Mastering the ‘New’ Keyword in JavaScript: A Comprehensive Guide
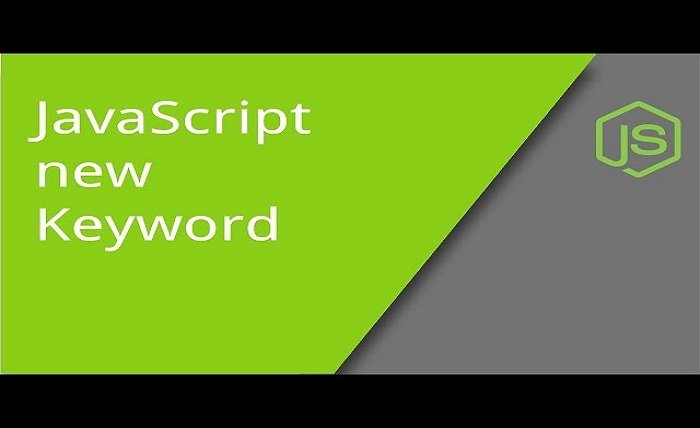
Introduction
The ‘new’ keyword in JavaScript is a fundamental aspect of object-oriented programming within the language. It plays a crucial role in creating new instances of objects, enabling developers to utilize both built-in and custom constructors effectively. This blog post delves into the intricacies of the ‘new’ keyword, providing a thorough understanding that will benefit both new and experienced JavaScript developers.
The ‘New’ Keyword
The ‘new’ keyword in JavaScript is used to create an instance of an object that has a constructor function. It prepares the environment for the object, setting up the prototype chain and ensuring that this
within the constructor points to the new object being created. Understanding how the ‘new’ keyword manipulates the execution context is essential for mastering JavaScript object creation.
How the ‘New’ Keyword Works
When you use the ‘new’ keyword in JavaScript, several steps are executed in the background:
- A new object is created.
- The object is linked to the constructor’s prototype.
- The constructor function is executed with
this
bound to the new object. - If the constructor returns an object, that object is returned; otherwise, the new object is returned.
These steps are critical in understanding how instances are created and how inheritance is facilitated in JavaScript.
Common Uses of the ‘New’ Keyword
The ‘new’ keyword is commonly used in JavaScript to:
- Create instances of custom objects.
- Implement inheritance by linking prototypes.
- Use built-in constructors like
Date
,RegExp
, and others.
Each use case demonstrates the flexibility and utility of the ‘new’ keyword in diverse JavaScript applications.
When to Use the ‘New’ Keyword
Deciding when to use the ‘new’ keyword can impact the architecture and readability of your code. It is generally recommended to use it when:
- You need objects with similar functionality and structure.
- You are implementing a pseudo-classical inheritance pattern.
- You want to encapsulate and manage state within an object.
Using the ‘new’ keyword appropriately is crucial for maintaining clean and manageable code in larger JavaScript projects.
The ‘New’ Keyword with ES6 Classes
With the introduction of ES6, the ‘new’ keyword has also found its place in class instantiation. ES6 classes are syntactic sugar over JavaScript’s existing prototype-based inheritance and require the ‘new’ keyword to create instances. Understanding this relationship helps in transitioning from traditional functions to class-based syntax.
Best Practices for Using the ‘New’ Keyword
Best practices for using the ‘new’ keyword in JavaScript include:
- Always using it with constructor functions to avoid unintended global variables.
- Naming constructor functions with an uppercase first letter to distinguish them from regular functions.
- Using the
instanceof
operator to check the type of the created object.
Following these guidelines ensures that the ‘new’ keyword is used effectively and consistently across different JavaScript projects.
Common Pitfalls and How to Avoid Them
Common pitfalls when using the ‘new’ keyword include:
- Forgetting to use the ‘new’ keyword with a constructor, leading to global contamination.
- Misunderstanding the prototype chain, resulting in inefficient code.
- Overusing the ‘new’ keyword, which can lead to overly complex structures that are difficult to maintain.
Awareness and careful planning can mitigate these issues, enhancing both code performance and maintainability.
The ‘New’ Keyword in Advanced JavaScript Patterns
In advanced JavaScript design patterns, such as the Singleton pattern or Factory functions, the ‘new’ keyword plays a pivotal role. These patterns often control the creation of instances and can leverage the ‘new’ keyword to enforce certain constraints or optimizations.
Alternatives to the ‘New’ Keyword
While the ‘new’ keyword is powerful, modern JavaScript offers alternatives like Object.create for situations where more control over the prototype chain is needed without invoking a constructor. Comparing these alternatives provides a rounded understanding of object creation in JavaScript beyond the ‘new’ keyword.
‘New’ Keyword Under the Hood
To truly master the ‘new’ keyword, one must understand what happens under the hood in the JavaScript engine. This involves the creation of execution contexts, handling of prototypes, and the this
binding process. Such knowledge is invaluable for debugging and optimizing JavaScript code.
Conclusion
The ‘new’ keyword is a cornerstone of object-oriented programming in JavaScript, essential for creating structured, reusable, and encapsulated code. By mastering its usage, developers can effectively design and maintain complex applications. Remember, the power of the ‘new’ keyword extends beyond syntax; it’s about creating a foundation for robust JavaScript applications.
FAQs
1. What does the ‘new’ keyword actually do in JavaScript? The ‘new’ keyword creates a new object, sets up the prototype chain, binds this
to the new object in the constructor, and determines the return value based on the constructor’s actions.
2. Can I use the ‘new’ keyword with arrow functions? No, arrow functions cannot be used as constructors and will throw an error if used with the ‘new’ keyword because they do not have their own this
context.
3. What is the difference between using ‘new Object()’ and object literals in JavaScript? Using ‘new Object()’ creates an object explicitly through a constructor, while object literals provide a shorthand syntax for creating objects and are more idiomatic in JavaScript.
4. How does the ‘new’ keyword affect the prototype chain? The ‘new’ keyword sets the prototype of the new object to the prototype property of the constructor function, facilitating prototype-based inheritance.
5. What are some common mistakes to avoid when using the ‘new’ keyword? Common mistakes include forgetting to use the ‘new’ keyword with constructors, leading to unintended global variables and mismanagement of the prototype chain, which can affect performance and lead to bugs.