Mastering the Golang ‘new’ Keyword: A Comprehensive Guide
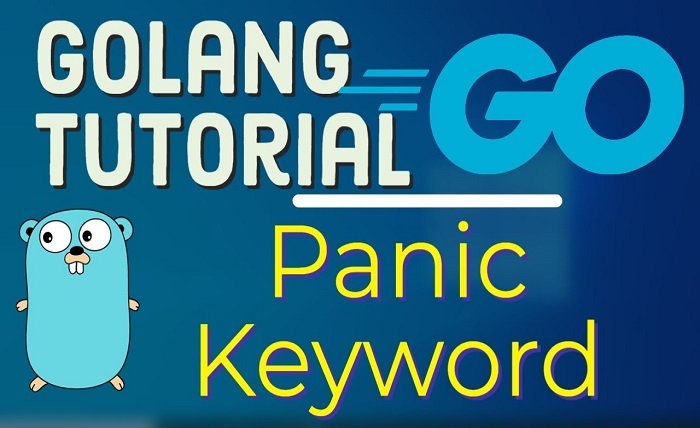
Introduction
The Golang ‘new’ keyword is a fundamental aspect of memory management in Go programming. It plays a crucial role in allocating memory for different types of data structures. This blog post delves into the mechanics of the ‘new’ keyword, illustrating its uses, benefits, and how it compares to alternative methods like composite literals and the ‘make’ keyword.
Memory Allocation in Go
Before we can fully appreciate the ‘new’ keyword, it’s essential to understand how memory allocation works in Go. The ‘new’ keyword allocates memory for a variable and returns a pointer to it. This section explains the internal workings and how it affects your Go programs.
Syntax and Basic Usage of ‘new’
The ‘new’ keyword in Go is simple in syntax but powerful in functionality. Here, we explore the basic usage of ‘new’, including code examples to demonstrate how to initialize different data types and structures.
‘new’ vs. ‘make’
Golang provides two built-in functions to allocate memory: ‘new’ and ‘make’. This section compares ‘new’ and ‘make’, highlighting their differences, appropriate use cases, and how to choose between them based on the needs of your application.
Common Pitfalls with ‘new’
While ‘new’ is straightforward to use, certain pitfalls can lead to bugs or inefficient code. We discuss common mistakes developers make when using the ‘new’ keyword in Go and how to avoid them.
Advanced Techniques Using ‘new’
For seasoned Go developers, ‘new’ can be used in more advanced scenarios. This part covers sophisticated techniques, including integrating ‘new’ with interfaces and other Go features to build robust applications.
Best Practices for Using ‘new’
To maximize the effectiveness of the ‘new’ keyword in Go programming, adhering to best practices is essential. This section outlines key strategies and coding standards to ensure optimal use of ‘new’ in your projects.
Performance Implications of Using ‘new’
Using ‘new’ can have implications on the performance of Go applications. We analyze performance considerations, benchmark comparisons, and how to mitigate any negative impacts when using ‘new’.
Integrating ‘new’ with Go Routines
Concurrency is a strength of Go, and understanding how to use ‘new’ with go routines is crucial for developing concurrent applications. This part explores the synchronization and potential pitfalls when using ‘new’ in multi-threaded environments.
‘new’ in the Context of Go’s Garbage Collection
Go’s garbage collector plays a significant role in how memory management is handled in the background. Here, we examine how ‘new’ interacts with garbage collection and what developers need to know to manage memory effectively.
Common Use Cases and Examples
To solidify understanding, this section provides practical examples and common use cases where the ‘new’ keyword is effectively employed in real-world Go applications.
The Future of ‘new’ in Go
Looking forward, we speculate on the developments and potential changes to the ‘new’ keyword in future versions of Go. This discussion includes insights from Go’s developers and what programmers might expect.
Conclusion
The Golang ‘new’ keyword is a powerful tool for memory management that, when used correctly, can enhance the performance and readability of your applications. By understanding and applying the guidelines and techniques discussed, developers can effectively utilize ‘new’ to its full potential.
FAQs
- What is the primary use of the Golang ‘new’ keyword?
- The ‘new’ keyword in Go is used for memory allocation. It initializes a zero-valued variable and returns a pointer to it.
- How does ‘new’ differ from ‘make’ in Go?
- ‘new’ returns a pointer to a new, zero-valued instance of a type, while ‘make’ is used only for slices, maps, and channels, and it returns an initialized (not zero-valued) instance of those types.
- Can ‘new’ be used with any data type?
- Yes, the ‘new’ keyword can be used with any type, including built-in types, struct types, and pointer types.
- Is it better to use ‘new’ or composite literals in Go?
- The choice depends on the context. Composite literals are generally more versatile and readable, but ‘new’ can be useful when you need a pointer to a zeroed structure.
- What are the best practices for using ‘new’ in high-performance applications?
- Best practices include understanding when to use ‘new’ versus other initialization methods, considering the impact on garbage collection, and ensuring proper synchronization in concurrent applications.