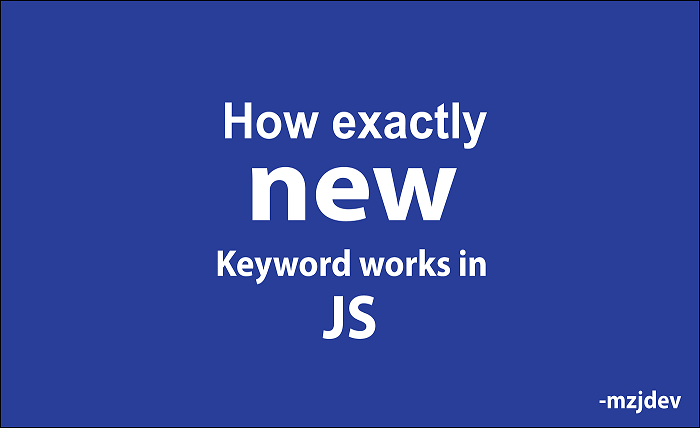
In JavaScript, the ‘new’ keyword plays a crucial role in object-oriented programming by allowing developers to create instances of user-defined objects and built-in object types. This blog post will delve deep into the nuances of the ‘new’ keyword, providing a clear understanding of its functionality and best practices for its use in various programming scenarios.
The ‘new’ Keyword
The ‘new’ keyword is used in JavaScript to create a new instance of an object that has a constructor function. It creates a fresh object, sets the prototype of this object to the object defined by the constructor’s prototype
property, and then calls the constructor function on the newly created object, allowing this object to be initialized.
How the ‘new’ Keyword Works
When you use the ‘new’ keyword, several steps happen under the hood:
- A new empty object is created.
- The object is linked to the constructor function’s prototype.
- The constructor function is executed with
this
bound to the new object. - If the constructor function returns an object, that object is returned; otherwise, the new object created in step one is returned.
The Role of Constructor Functions
Constructor functions are like blueprints for objects. When combined with the ‘new’ keyword, they define the properties and methods that the new objects will have. For instance, a Car
constructor function can be used to create multiple car objects with properties like make
, model
, and year
.
Differences Between Object Creation Methods
There are several ways to create objects in JavaScript:
- Object literals
- Factory functions
- The ‘new’ keyword with constructors Understanding when and why to use the ‘new’ keyword over other methods is key to mastering JavaScript object creation.
Common Pitfalls with the ‘new’ Keyword
A common mistake when using the ‘new’ keyword is forgetting to use it when calling a constructor. This can lead to unexpected behavior, as the this
keyword inside the constructor will not point to a new object, but rather to the global object (in non-strict mode) or be undefined
(in strict mode).
Best Practices for Using ‘new’
To maximize the effectiveness and readability of your code:
- Always use the ‘new’ keyword when calling constructors.
- Name constructor functions with a capital letter to distinguish them from regular functions.
- Ensure constructors are designed to work only with the ‘new’ keyword.
‘new’ Keyword in ES6 Classes
In ES6, the ‘new’ keyword becomes even more important with the introduction of classes. Classes in JavaScript are primarily syntactical sugar over the existing prototype-based inheritance and need the ‘new’ keyword to create instances.
Performance Considerations
Using the ‘new’ keyword can have implications for performance, especially in high-load applications. It is important to benchmark your applications if you suspect that object creation might be a bottleneck.
Alternatives to the ‘new’ Keyword
Frameworks and libraries like React sometimes discourage the direct use of the ‘new’ keyword in favor of factory functions or other creation patterns. Understanding these can help in adopting modern JavaScript frameworks more effectively.
‘new’ Keyword Under the Hood
For those interested in the deeper mechanics, the ‘new’ keyword’s behavior is defined in the ECMAScript specification. It involves intricate steps of object creation, prototype linking, and constructor execution.
Advanced Usage of ‘new’
In advanced JavaScript, the ‘new’ keyword can be paired with higher-order functions and closures to create powerful and encapsulated modules.
Conclusion
The ‘new’ keyword is a fundamental aspect of JavaScript, crucial for effective object-oriented programming. By understanding and correctly utilizing this keyword, developers can harness the full power of JavaScript to create well-structured, efficient, and encapsulated applications.
FAQs
- What happens if I forget to use the ‘new’ keyword with a constructor? Forgetting to use ‘new’ results in the
this
keyword not being bound to a new object, which can lead to unexpected global modifications or errors in strict mode. - Can I use the ‘new’ keyword with arrow functions? No, arrow functions do not have a
this
binding and cannot be used as constructors. If you try to use ‘new’ with an arrow function, JavaScript will throw an error. - Is the ‘new’ keyword necessary with built-in constructors like
Object()
orArray()
? While not strictly necessary (since these constructors return objects when called without ‘new’), using ‘new’ can make your intentions clearer and your code more consistent. - How does the ‘new’ keyword affect memory usage? Each use of the ‘new’ keyword creates a new object, which consumes memory. However, JavaScript engines are optimized to handle such creations efficiently.
- Are there any recent changes to how the ‘new’ keyword works in JavaScript? The fundamental operation of ‘new’ has not changed, though modern JavaScript versions (like ES6 with classes) provide new contexts and patterns for its use.